In two streams I managed to make a simple clone of Asteroids. As has been my trend lately I used the DirectX wrapper for C# called SharpDX to handle the graphics.
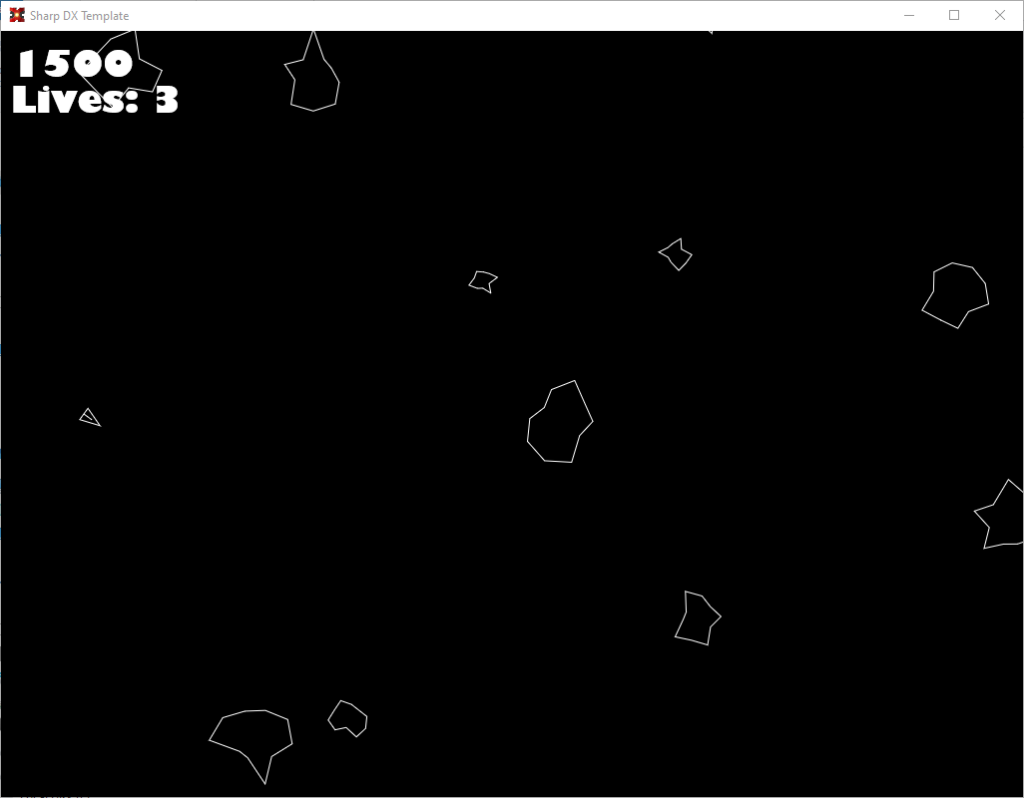
I published the source code on my Github/CrookedFingerGuy with a stand alone .exe file.
One thing I did off stream to help me understand the whole rotating polygons situation was I made a toy program. That program helped me learn to draw functions and rotate vectors using SharpDX. I also related the unit circle and the the sine and cosine functions.

The interesting bit of cod e for this project was as follows:
//unit vector on unit circle
solidColorBrush.Color= new RawColor4(0.7529412f, 0.7529412f, 0.7529412f, 1.0f);
d2dRenderTarget.DrawLine(new Vector2(tx,ty),new Vector2(direction.X*magnitude+tx,direction.Y*magnitude+ty),solidColorBrush);
d2dRenderTarget.DrawEllipse(new Ellipse(new RawVector2(tx,ty),magnitude,magnitude), solidColorBrush);
//sine component of unit vector
solidColorBrush.Color = new RawColor4(0.5019608f, 0.5019608f, 1f, 1.0f);
d2dRenderTarget.DrawLine(new Vector2(tx + direction.X * magnitude, ty),
new Vector2(direction.X * magnitude + tx, direction.Y * magnitude + ty), solidColorBrush);
//cosine component of unit vector
solidColorBrush.Color= new RawColor4(0.1960784f, 0.8039216f, 0.2431373f, 1.0f);
d2dRenderTarget.DrawLine(new Vector2(tx + direction.X * magnitude, ty), new Vector2(tx, ty), solidColorBrush);
//red dot on unit circle
solidColorBrush.Color= new RawColor4(1f, 0f, 0f, 1.0f);
d2dRenderTarget.FillEllipse(new Ellipse(new RawVector2(direction.X * magnitude + tx,
direction.Y * magnitude + ty), 3f, 3f), solidColorBrush);
//sin graph
sinXOffset = 50f;
sinYOffset = 375;
sinGraphWidth = magnitude*4;
sinGraphHeight = sinGraphWidth / 2;
solidColorBrush.Color = new RawColor4(0.7529412f, 0.7529412f, 0.7529412f, 1.0f);
d2dRenderTarget.DrawLine(new Vector2(sinXOffset, sinYOffset),
new Vector2(sinXOffset + sinGraphWidth, sinYOffset), solidColorBrush);
d2dRenderTarget.DrawLine(new Vector2(sinXOffset + sinGraphWidth / 2, sinYOffset - sinGraphHeight / 2),
new Vector2(sinXOffset + sinGraphWidth / 2, sinYOffset + sinGraphHeight / 2), solidColorBrush);
PathGeometry shape = new PathGeometry(d2dFactory);
GeometrySink sink = shape.Open();
float y = (float)(sinGraphWidth / 4 * Math.Sin((float)-Math.PI));
int x = 0;
Vector2 vect = new Vector2(x + sinXOffset, y + sinYOffset);
sink.BeginFigure(vect, FigureBegin.Hollow);
for (x = 0; x < sinGraphWidth; x++)
{
vect.X = (float)(x + sinXOffset);
vect.Y = (float)Math.Sin(Map((float)x, (float)0, (float)sinGraphWidth,
(float)-Math.PI, (float)Math.PI)) * sinGraphHeight / 2 + sinYOffset;
sink.AddLine(vect);
}
sink.EndFigure(FigureEnd.Open);
sink.Close();
d2dRenderTarget.DrawGeometry(shape, solidColorBrush, 1f);
//sine component of unit vector
float sMapX = Map(rotation,-(float)(Math.PI),(float)(Math.PI),0, sinGraphWidth);
float sMapY=Map(direction.Y,-1,1,-sinGraphHeight / 2, sinGraphHeight / 2);
solidColorBrush.Color = new RawColor4(0.5019608f, 0.5019608f, 1f, 1.0f);
d2dRenderTarget.DrawLine(new Vector2(sMapX+ sinXOffset, sinYOffset),
new Vector2(sMapX+ sinXOffset, sMapY + sinYOffset), solidColorBrush);
//cosine graph
cosXOffset = 50f;
cosYOffset = 600;
cosGraphWidth = magnitude*4;
cosGraphHeight = cosGraphWidth/2;
solidColorBrush.Color = new RawColor4(0.7529412f, 0.7529412f, 0.7529412f, 1.0f);
d2dRenderTarget.DrawLine(new Vector2(cosXOffset,cosYOffset),
new Vector2(cosXOffset+ cosGraphWidth, cosYOffset), solidColorBrush);
d2dRenderTarget.DrawLine(new Vector2(cosXOffset+ cosGraphWidth/2, cosYOffset- cosGraphHeight/2),
new Vector2(cosXOffset + cosGraphWidth/2, cosYOffset+ cosGraphHeight/2), solidColorBrush);
shape = new PathGeometry(d2dFactory);
sink = shape.Open();
y = (float)(cosGraphWidth / 4 * Math.Cos((float)-Math.PI));
x = 0;
vect = new Vector2(x+cosXOffset,y+cosYOffset);
sink.BeginFigure(vect, FigureBegin.Hollow);
for(x=0;x<cosGraphWidth;x++)
{
vect.X = (float)(x+cosXOffset);
vect.Y = (float)Math.Cos(Map((float)x, (float)0, (float)cosGraphWidth,
(float)-Math.PI, (float)Math.PI))*cosGraphHeight/2+cosYOffset;
sink.AddLine(vect);
}
sink.EndFigure(FigureEnd.Open);
sink.Close();
d2dRenderTarget.DrawGeometry(shape, solidColorBrush, 1f);
//cosine component of unit vector
float cMapX = Map(rotation, -(float)(Math.PI), (float)(Math.PI), 0, sinGraphWidth);
float cMapY = Map(direction.X, -1, 1, -sinGraphHeight / 2, sinGraphHeight / 2);
solidColorBrush.Color = new RawColor4(0.1960784f, 0.8039216f, 0.2431373f, 1.0f);
d2dRenderTarget.DrawLine(new Vector2(cMapX + cosXOffset, cosYOffset),
new Vector2(cMapX + cosXOffset, cMapY + cosYOffset), solidColorBrush);
solidColorBrush.Color = Color.White;
d2dRenderTarget.DrawText("Radians: "+rotation.ToString("0.00")+" Degrees: "+(rotation*180/Math.PI).ToString("0."),
TestTextFormat, TestTextArea, solidColorBrush);
solidColorBrush.Color = new RawColor4(0.7529412f, 0.7529412f, 0.7529412f, 1.0f);
asteroid.DrawAsteroid(d2dRenderTarget, d2dFactory, solidColorBrush);